Introduction
Welcome to my Project Portfolio which provides you with an overview of my technical skills in the writing of documentation, coding and other contributions to my team project, MediTabs, for a Software Engineering module in the National University of Singapore (NUS).
About the project
The team project involved improving an existing Addressbook application for users who prefer command line interfaces (CLI). My team and I chose to morph it into a medicine inventory management application called MediTabs. The goal is to streamline the medicine inventory management workflow in clinics by enabling pharmacists to keep track and manage medicine inventory easily; export the data for easier reference and data analysis; and print medicine labels containing essential medicine information.
I am the team leader and in charge of designing and implementing the export
feature.
This document uses the following labels and formatting:
Label/Formatting | Description | ||
---|---|---|---|
|
Indicates command, format or code. |
||
Information to take note. |
|||
Important information. |
|||
Indicates potential error message. |
Summary of contributions
This section highlights my documentation, coding and other noteworthy contributions to my team project.
-
Major enhancement: Added
export
command support-
What it does: Allows the user to export the current medicine inventory data shown in the Graphical User Interface (GUI) to a spreadsheet file which is supported by commonly used spreadsheet applications such as Microsoft Excel.
-
Justification: Allows the user to save the data so that it can be printed, organised or for advanced data analysis using a spreadsheet application such as Microsoft Excel. This is especially useful for the user, superior or supplier’s reference.
-
Highlights: This enhancement requires an indepth understanding of how each major components work and the methods available as it involves retrieving of data from the GUI and parsing the retrieved data. Despite using the Opencsv library, the implementation was still challenging as it required using methods from different components, parsing and organising the data such that the exported file contains only the essential information for easier reference.
-
Credits: Opencsv Team
-
-
Minor enhancement: As there are currently no third party libraries available which validate file name such that it adheres to the file naming conventions of the major operating systems (OS), Windows, Linux and macOS, I have created a
FileName
class which implements this feature to complement theexport
feature and is also used by thelabel
feature. It requires an indepth knowledge of the different file naming conventions used in the various OS and also numerous testing as I discovered that some file name conventions were not documented and not allowed to be used as file names. -
Code contributed: Project Code Dashboard
-
Other contributions:
-
Project management:
-
Set up team project GitHub repository including setting up the issue tracker, permissions, project page, milestones and labels.
-
Managed releases
v1.1
-v1.4
(9 releases) on GitHub
-
-
Documentation:
-
Added Introduction sections to both User Guide and Developer Guide: #115, #140
-
Added Frequently Asked Question (FAQ) and File Naming Convention sections to User Guide: #123, #135
-
Added Validation of File Name section to Developer Guide which includes code snippet for reference on how to take advantage of the
FileName
class provided: #140 -
Updated stylesheet to Portable Document Format (PDF) printer friendly format: #150
-
Updated User Guide’s Quick Start section and Developer Guide’s Setting Up section to only allow Java version
9
and JDK9
including references to the reason for the change: #117, #123 -
Updated User Guide Quick Start section with an additional step on how users can seek assistance: #115
-
Updated Storage component class diagram of Developer Guide to match our project: #79
-
Updated Developer Guide’s Instructions for Manual Testing section to include instructions for testing the
export
command: #197
-
-
Community:
-
Tools:
-
Contributions to the User Guide
To showcase my ability to write documentation which targets end users, my contributions to the User Guide are shown below.
Exporting as CSV file format : export
Exports the current medicine inventory data shown in the GUI as CSV file format.
Format: export [FILE_NAME]
Medicines without any batches are not included in the exported CSV file as they would not have any useful information such as the quantity and expiry dates which are important in the keeping track of your medicine inventory. This is by design, as our team believes that the exported CSV file should only provide useful detailed information. |
Examples:
-
export
Exports the current medicine inventory data shown in the GUI to a CSV file which has a default file name format{Date of export}_{Time of export}
. e.g.18_Mar_2019_10_28_00
-
export example
Exports the current medicine inventory data shown in the GUI to a CSV file which has the filenameexample
.
If a file with the specified file name already exists in the default exported folder, the file would not be exported and an error message "Could not export data to csv file: {Specified File Name} already exists in "exported" directory"
|
A sample image of how the medicine inventory data in the exported CSV file is organised:
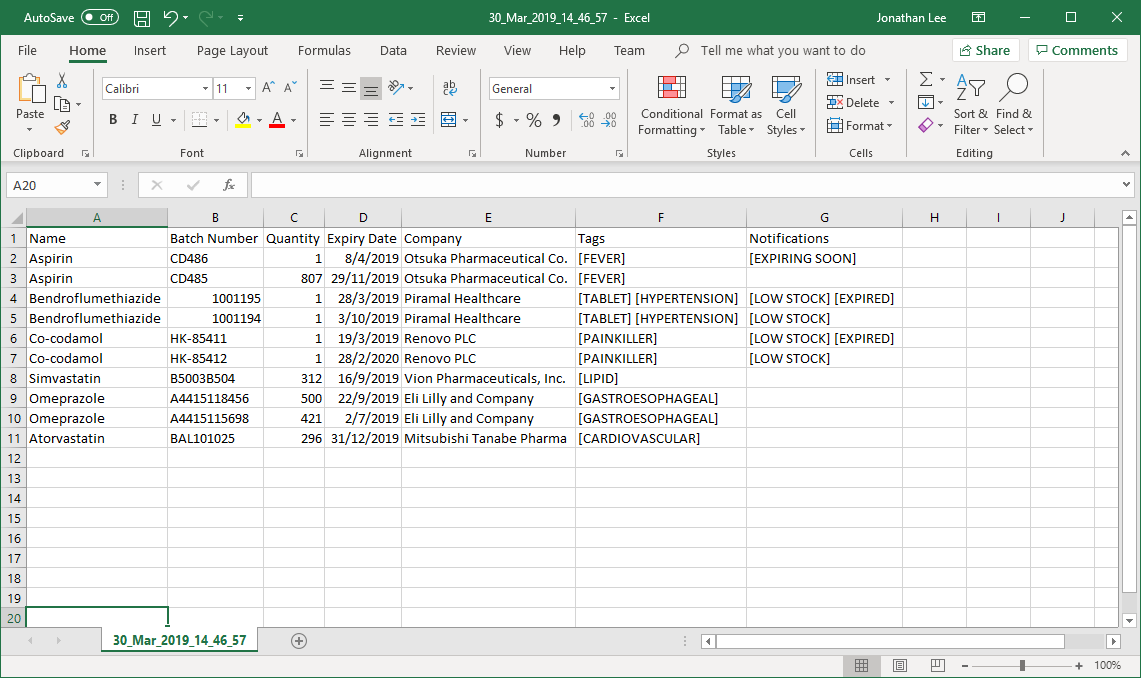
From the image above, you can observe that the exported CSV file only contains essential information such as the medicine batches, quantity and expiry date for each batch of medicine and which medicines are low in stock, expiring soon or have expired for easier reference. |
Integration Addon Support
You can download and install integration addons created for MediTabs such as addons which allows MediTabs to integrate with different third party services which your clinic might currently be using.
Format: install an/NAME_OF_ADDON
Only integration addons that have been verified by our team can be downloaded and installed in MediTabs. This is to ensure that the addons are secure and have no malicious code due to the increasing cybersecurity threat. |
Benefits of integration addon support are as follows:
-
Provides greater flexibility as as you might choose to integrate with different third-party services which are currently used by your clinic, to allow for easier transition between services.
-
Allows you to customise MediTabs with features that your clinic requires without any unnecessary features which might not be relevant to your clinic’s workflow.
An example of an integration addon which would be supported by MediTabs:
-
Singapore’s Health Sciences Authority (HSA) - To integrate the Singapore HSA’s medical database with MediTabs which allows easy retrieval of important information such as when medicine is requested to be recalled by the HSA.
This feature can be improved to support medical databases of different countries. |
Contributions to the Developer Guide
To showcase my ability to write technical documentation which targets developers and my technical knowledge on my project contributions, my contributions to the Developer Guide are shown below.
Exporting as CSV file format
This section provides you with an overview of how exporting as Comma-separated values (CSV) file format is implemented in MediTabs and the design considerations made by our team with regards to its implementation.
Current Implementation
The exporting as CSV file format mechanism is facilitated by CsvWrapper
.
It is built on top of the Opencsv
Java CSV parser library, licensed under the Apache 2 OSS License
, so that it integrates with our product. This is done by providing additional operations to support exporting the current medicine inventory data shown in the GUI to CSV file format.
There are many additional operations added in CsvWrapper
but we will only list the key operations which are the main drivers of the overall implementation of the feature for easier reference and understanding.
The key operations are as follows:
-
CsvWrapper#export()
— Export the current medicine inventory data shown in the GUI to CSV file format. -
CsvWrapper#createCsvFile(String csvFileName)
— Creates a CSV file with the file name based on thecsvFileName
input. The file is created in the defaultexported
directory which is located in the same directory as our product application.If the default exported
directory is not found, it will be automatically created. -
CsvWrapper#writeDataToCsv(List currentGuiList)
— Writes the current medicine inventory data shown in the GUI to the CSV file created by theCsvWrapper#createCsvFile(String csvFileName)
operation.
Out of the three key operations stated above, only CsvWrapper#export() is a public operation available for use by other components. It acts as the main interface which other components use to interact with CsvWrapper in order to integrate exporting to CSV file format feature into their own implementation.
|
Given below is a sequence diagram overview of how these 3 key operations behave when the user executes the export
command in order to export the current medicine inventory data shown in the GUI to CSV file format:
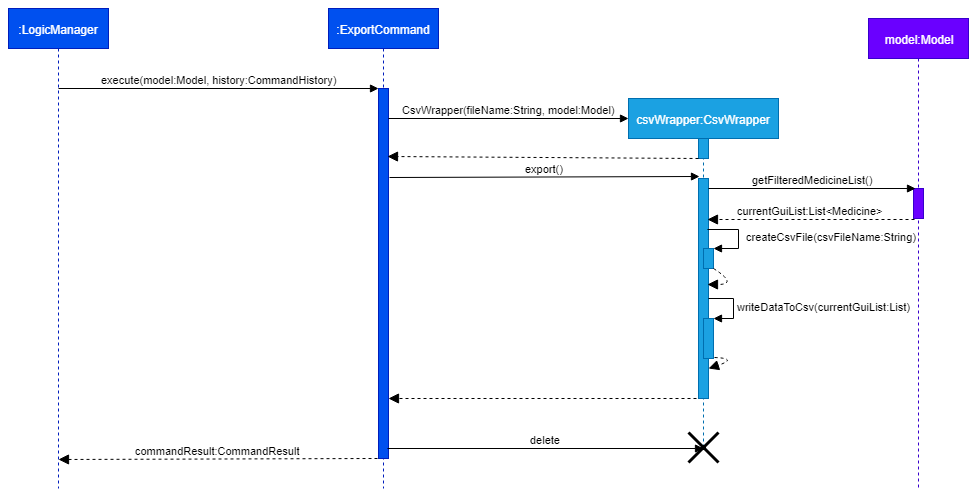
Given below is a brief description of how the exporting as CSV file format mechanism behaves as shown in the sequence diagram above:
Step 1: When the user executes the export
command, assuming parsing of the command line arguments have already been completed, the ExportCommand#execute()
operation is called.
Step 2: The ExportCommand#execute()
operation initialises the CsvWrapper
with the file name of the CSV file to be stored and the current model instance as its input parameters.
Step 3: The ExportCommand#execute()
operation then calls CsvWrapper#export()
operation which is the first key operation implementing the export to CSV file format feature.
Step 4: The CsvWrapper#export()
operation retrieves the current medicine inventory data shown in the GUI by calling the Model#getFilteredMedicineList()
operation.
Step 5: After retrieving the data, the CsvWrapper#createCsvFile(String csvFileName)
operation, which is the second key operation, is called. It creates an empty CSV file with the input file name in the default exported
directory.
If a CSV file with the input file name already exists in the exported directory, a "Could not export data to csv file: {Input File Name} already exists in "exported" directory" exception will be shown in the ResultDisplay panel of the GUI as a CommandException is thrown and the exporting process will stop executing. In other words, the current medicine inventory data shown in the GUI would not be exported.
|
Step 6: After the empty CSV file is created, the CsvWrapper#writeDataToCsv(List currentGuiList)
, which is the third key operation, is called to process the current medicine inventory data retrieved earlier in Step 4 and writes to the CSV file in an organised format for easier reference by the users of the exported CSV file.
Step 7: The current medicine inventory data shown in the GUI is exported successfully to CSV file format.
Step 8: The ExportCommand#execute()
operation returns a CommandResult
which shows the current list is exported to a CSV file with the input file name.
The following activity diagram summarizes what happens when a user executes the export
command:
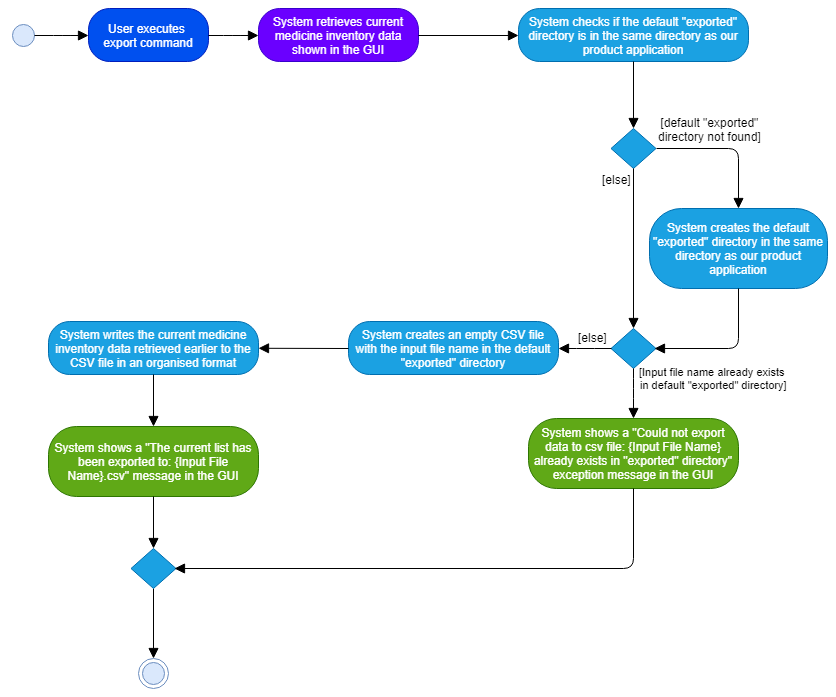
Design Considerations
Aspect: How exporting as CSV file format executes
-
Alternative 1 (current choice): Exports the current medicine inventory data shown in the GUI to CSV file format using the
export
command.-
Pros: Easy to implement and users can preview the data before exporting.
-
Cons: May have performance issues in terms of memory usage.
-
-
Alternative 2: Individual commands can add an additional
export
parameter to support exporting as CSV file format.-
Pros: Users can export directly through individual commands which support the additional
export
parameter (e.g. Thefind
command with its additionalexport
parameter set to true, exports the filtered medicine inventory data immediately without having to retrieve fromModel#getFilteredMedicineList()
operation). -
Cons: We must ensure that the implementation and integration of the exporting to CSV file of each individual commands are correct. Furthermore, users are not able to preview the data before exporting.
-
Aspect: Data structure/Algorithm/Implementation to support the export
command
-
Alternative 1 (current choice): Iterate through each of the medicine in the list retrieved from
Model#getFilterMedicineList()
operation to build the structure in which the data is organised when exported to CSV file format.-
Pros: Easy for developers to understand, especially for those who want to modify the way in which the data is organised when exported to CSV file format but have no prior knowledge on
Opencsv
Java CSV parser library. -
Cons: The time complexity of the algorithm is O(n) and might not be as efficient especially when a large amount of data is involved. Furthermore, it does not take full advantage of the more advanced features provided by the
Opencsv
Java CSV parser library.
-
-
Alternative 2: Use
Opencsv
Java CSV parser library’sStatefulBeanToCsvBuilder
operation for building the structure in which the data is organised from the list retrieved using theModel#getFilterMedicineList()
operation when exporting to CSV file format.-
Pros: Does not require iterating through the list and convert it to a String Array as we can use the library’s
StatefulBeanToCsvBuilder
operation to build the structure from the list by passing the list as a parameter to the operation. Furthermore, the formatting process can be automated using the operation. It is also more efficient in terms of performance according to the library’s documentation if the ordering of the data exported is not a concern to the developer. -
Cons: Requires prior knowledge on the way in which the library’s
StatefulBeanToCsvBuilder
operation works. If a developer wants to modify the data exported to be ordered in a specific format, it requires knowledge on the library’sMappingStrategy
related operations which may be complicated for developers new to the library.More information on Opencsv
library’sStatefulBeanToCsvBuilder
operation can be found in the library’s documentation.
-